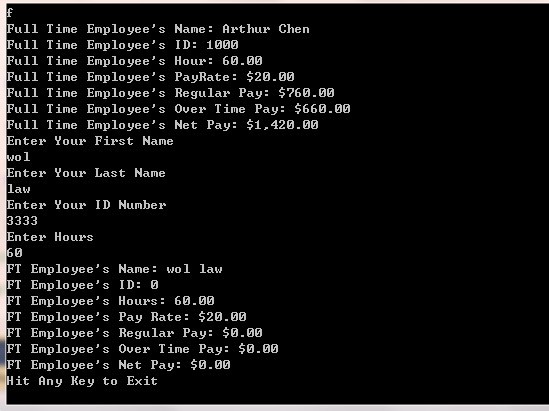
我不是很懂为什么会出现这个情况。
我的代码写的都差不多,至少我看了几遍,hour和ID都基本一样。但我真心不知道,为什么ID不会显示出数值来,还有工资也是。
接来下的代码有点长,我是完全基本完全复制了。 还请见谅。
//full Time Employee Class
namespace EmPloyeeChen
{
public class FullTimeEmployee //object
{
private string FEmployeeFName, FEmployeeLName;
private double FEmployeeID;
private double FEmployeeWorkHour;
private const double FEmployeePayRate = 20.00;
private double GrossPay, RegPay, OTPay;
//No Args Constructor, Default, Silly
public FullTimeEmployee()
{
FEmployeeFName = "Arthur";
FEmployeeLName = "Chen";
FEmployeeID = 1000;
FEmployeeWorkHour = 60;
RegPay = calcRegPay();
OTPay = CalOTPay();
}
//Constructor
public FullTimeEmployee(string FFName, string FLName, double FID, double FHours)
{
FEmployeeFName = FFName;
FEmployeeLName = FLName;
FID = FEmployeeID;
FEmployeeWorkHour = FHours;
//FEmployeePayRate = FPRate;
}
//Accessor Methods
//get name
public string GetFName()
{
return FEmployeeFName + " " + FEmployeeLName;
}
//get ID
public double getID()
{
return FEmployeeID;
}
//get Payrate
public double getPayRate()
{
return FEmployeePayRate;
}
//get hours
public double getHours()
{
return FEmployeeWorkHour;
}
//**********************************
//******Calculate GrossPay**********
//**********************************
//Calculate RegularPay
private double calcRegPay()
{
double RegPay;
if (FEmployeeWorkHour >= 0 && FEmployeeWorkHour <= 38)
{
RegPay = FEmployeeWorkHour * FEmployeePayRate;
}
else
{
RegPay = 38 * FEmployeePayRate;
}
return RegPay;
}
//get Regular Pay
public double getRegPay()
{
return RegPay;
}
//calculate OverTime Pay
private double CalOTPay()
{
double OTPay;
if (FEmployeeWorkHour >= 0 && FEmployeeWorkHour <= 38)
{
OTPay = 0;
}
else
{
OTPay = (FEmployeeWorkHour - 38) * FEmployeePayRate * 1.5;
}
return OTPay;
}
//get OTPay
public double getOTPay()
{
return OTPay;
}
//get Gross Pay
public double getGrossPay()
{
return GrossPay = RegPay + OTPay;
}
} //end FullTimeEployee Class
} //end Name Space
//EmployeeDriver
public static void CreateFullTime()
{ //get output for default constructor
FullTimeEmployee myFT;
myFT = new FullTimeEmployee();
//outputing with formatted strings
Console.WriteLine("Full Time Employee's Name: " + myFT.GetFName());
Console.WriteLine("Full Time Employee's ID: " + myFT.getID());
Console.WriteLine("Full Time Employee's Hour: " + myFT.getHours().ToString("n2"));
Console.WriteLine("Full Time Employee's PayRate: " + myFT.getPayRate().ToString("C2"));
Console.WriteLine("Full Time Employee's Regular Pay: " + myFT.getRegPay().ToString("C2"));
Console.WriteLine("Full Time Employee's Over Time Pay: " + myFT.getOTPay().ToString("C2"));
Console.WriteLine("Full Time Employee's Net Pay: " + myFT.getGrossPay().ToString("C2"));
//some temporary variables
string LN = " ", FN = " ";
double FId = -8888;
double FHrs = -10;
bool checkFN=false;
bool checkLN = false;
bool CheckID = false;
bool CheckHour = false;
//input the correct ID number
do //do loop
{
try
{
Console.WriteLine("Enter Your ID Number");
string inputid = Console.ReadLine();
FId = double.Parse(inputid); //catch the input
// FId = double.Parse(Console.ReadLine());
}
catch (FormatException fmte)
{
Console.WriteLine(fmte.Message);
}
if (FId >= 1001 && FId <= 9999)
{
CheckID = true; //help me get out, since ID is in the range
}
else
{
Console.WriteLine("ID Number Must Be 4 Digit Number "
+ "and greater than 1000");
CheckID = false; //help me to stay in that, since what type is wrong
}
} while (!CheckID); //keep going as long as If is false
//Enter workhours
do
{
try
{
Console.WriteLine("Enter Hours");
string inputhr = Console.ReadLine();
FHrs = double.Parse(inputhr); //convert inputhr string to double
}
catch (FormatException fmte)
{
Console.BackgroundColor = ConsoleColor.Red;
Console.WriteLine("Dude\n"
+ "It is NOT a Legic Number!!" + fmte.Message);
}
if (FHrs > 0 && FHrs <= 65)
{
CheckHour = true; //hlep me out
}
else
{
Console.WriteLine("Please Enter a Positive Number\n"
+ "And the maxmium number is 65!!!");
CheckHour = false;
}
} while (!CheckHour); //keep going as long as If is false
FullTimeEmployee YrFT;
YrFT = new FullTimeEmployee(FN, LN, FId, FHrs);
Console.BackgroundColor = ConsoleColor.Black;
//outputing
Console.WriteLine("FT Employee's Name: " + YrFT.GetFName());
Console.WriteLine("FT Employee's ID: " + YrFT.getID());
Console.WriteLine("FT Employee's Hours: " + YrFT.getHours().ToString("n2"));
Console.WriteLine("FT Employee's Pay Rate: " + YrFT.getPayRate().ToString("c2"));
Console.WriteLine("FT Employee's Regular Pay: " + YrFT.getRegPay().ToString("C2"));
Console.WriteLine("FT Employee's Over Time Pay: " + YrFT.getOTPay().ToString("C2"));
Console.WriteLine("FT Employee's Net Pay: " + YrFT.getGrossPay().ToString("c2"));
} //end class
}
} //end name sapce
我看了几遍,没找到出错的地方啊。
真心不懂哪里出错了。