我用UDP写了一个群聊软件的例程,在自己电脑上也能运行,但是release发布到其他电脑上一直报错,
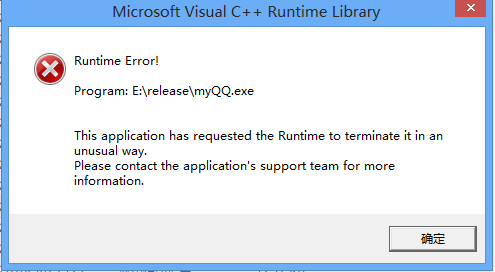
之后我尝试了在其他电脑上直接运行源码,但是发送消息的时候也只有我的电脑上能看到消息更新,对方电脑上只有自己的消息,不能接收我的消息,求指教!
附界面图和源码如下:
drawer.h----------------------------------------------------------------------------------------------------------
#ifndef DRAWER_H
#define DRAWER_H
#include<QToolBox>
#include<QToolButton>
#include<QTextEdit>
#include<QVBoxLayout>
#include<QPushButton>
#include"widget.h"
class Drawer : public QToolBox
{
Q_OBJECT
public:
Drawer(QWidget *parent=0, Qt::WindowFlags f=0);
private:
//main window
QToolButton *toolBtn1;
QToolButton *toolBtn2;
QToolButton *toolBtn3;
QToolButton *toolBtn4;
QToolButton *toolBtn5;
QToolButton *toolBtn6;
QToolButton *toolBtn7;
QToolButton *toolBtn8;
QToolButton *toolBtn9;
Widget *chatWidget1;
Widget *chatWidget2;
private slots:
void showChatWidget1();
void showChatWidget2();
};
#endif // DRAWER_H
widget.h--------------------------------------------------------------------------------------------------------------
#ifndef WIDGET_H
#define WIDGET_H
#include <QWidget>
class QUdpSocket;
namespace Ui {
class Widget;
}
enum MsgType{Msg, UsrEnter, UsrLeft, FileName, Refuse};
class Widget : public QWidget
{
Q_OBJECT
public:
explicit Widget(QWidget *parent , QString usrname);
~Widget();
protected:
void usrEnter(QString usrname, QString ipaddr); //新用户加入
void usrLeft(QString usrname, QString time); //用户离开
void sndMsg(MsgType type, QString srvaddr=""); //广播UDP消息
void closeEvent(QCloseEvent *); //用户离开广播
QString getIP(); //获取IP地址
QString getUsr(); //获取用户名
QString getMsg(); //获取聊天信息
private:
Ui::Widget *ui;
QUdpSocket *udpSocket;
qint16 port;
QString uName;
private slots:
void processPendingDatagrams(); //接收UDP消息
void on_sendBtn_clicked();
void on_exitBtn_clicked();
};
#endif // WIDGET_H
drawer.cpp-------------------------------------------------------------------------------------------------------
#include"drawer.h"
#include<QGroupBox>
#include<QVBoxLayout>
Drawer::Drawer(QWidget *parent, Qt::WindowFlags f)
:QToolBox(parent,f)
{
//main window
setWindowTitle(tr("QQ2016"));
setWindowIcon(QPixmap(":/images/title.png"));
//first friend info
toolBtn1 = new QToolButton;
toolBtn1->setText(tr("周杨松")); //friend name 1
toolBtn1->setIcon(QPixmap(":/images/baoman.png"));
toolBtn1->setIconSize(QPixmap(":/images/baoman.png").size());
toolBtn1->setAutoRaise(true);
toolBtn1->setToolButtonStyle(Qt::ToolButtonTextBesideIcon);
//second friend info
toolBtn2 = new QToolButton;
toolBtn2->setText(tr("队长")); //friend name 2
toolBtn2->setIcon(QPixmap(":/images/kenan.png"));
toolBtn2->setIconSize(QPixmap(":/images/kenan.png").size());
toolBtn2->setAutoRaise(true);
toolBtn2->setToolButtonStyle(Qt::ToolButtonTextBesideIcon);
//layout
QGroupBox *groupBox = new QGroupBox;
QVBoxLayout *layout = new QVBoxLayout(groupBox);
layout->setMargin(20);
layout->setAlignment(Qt::AlignLeft);
layout->addWidget(toolBtn1);
layout->addWidget(toolBtn2);
layout->addStretch();
this->addItem((QWidget*)groupBox,tr("群成员"));
//connect
connect(toolBtn1,SIGNAL(clicked()),this,SLOT(showChatWidget1()));
connect(toolBtn2,SIGNAL(clicked()),this,SLOT(showChatWidget2()));
toolBtn1->setAttribute(Qt::WA_DeleteOnClose);
toolBtn2->setAttribute(Qt::WA_DeleteOnClose);
}
void Drawer::showChatWidget1()
{
chatWidget1 = new Widget(0,toolBtn1->text());
chatWidget1->setWindowTitle(toolBtn1->text());
chatWidget1->setWindowIcon(toolBtn1->icon());
//chatWidget1->resize();
chatWidget1->show();
chatWidget1->setAttribute(Qt::WA_DeleteOnClose);
}
void Drawer::showChatWidget2()
{
chatWidget2 = new Widget(0,toolBtn2->text());
chatWidget2->setWindowTitle(toolBtn2->text());
chatWidget2->setWindowIcon(toolBtn2->icon());
chatWidget2->show();
chatWidget2->setAttribute(Qt::WA_DeleteOnClose);
}
widget.cpp-----------------------------------------------------------------------------------------------------
#include "ui_widget.h"
#include "widget.h"
#include <qdatetime.h>
#include <QUdpSocket>
#include <QHostInfo>
#include <QMessageBox>
#include <QScrollBar>
#include <QDateTime>
#include <QNetworkInterface>
#include <QProcess>
#include <QTime>
Widget::Widget(QWidget *parent,QString usrname):
QWidget(parent)
,ui(new Ui::Widget)
{
ui->setupUi(this);
uName=usrname;
udpSocket=new QUdpSocket(this);
port=23232;
//QString Qport=port;
//ui->messagebox->setText("a"+port);
udpSocket->bind(port,QUdpSocket::ShareAddress | QUdpSocket::ReuseAddressHint);
connect(udpSocket,SIGNAL(readyRead()),this,SLOT(processPendingDatagrams()));
//connect(udpSocket,SIGNAL(readyRead()),this,SLOT(usrEnter()));
sndMsg(UsrEnter); //有用户进入,初始化
}
Widget::~Widget()
{
delete ui;
}
//UDP初始化
void Widget::sndMsg(MsgType type, QString srvaddr)
{
QByteArray data;
QDataStream out(&data, QIODevice::WriteOnly);
QString address=getIP();
//ui->messagebox->setText(address);
out<< type << getUsr();
//ui->messagebox->append(getUsr());
switch(type)
{
case Msg:
if(ui->send_message->toPlainText()=="") {
QMessageBox::warning(0,tr("警告"),tr("发送内容不能为空"),QMessageBox::Ok);
return;
}
out << address <<getMsg();
ui->messagebox->verticalScrollBar()->setValue(
ui->messagebox->verticalScrollBar()->maximum());
break;
case UsrEnter: { out<<address; break; }
case UsrLeft: break;
case FileName: break;
case Refuse: break;
}
udpSocket->writeDatagram(data,data.length(),QHostAddress::Broadcast,port);
}
//发送UDP广播
void Widget::processPendingDatagrams()
{
//ui->messagebox->setValue(udpSocket->hasPendingDatagrams());
while(udpSocket->hasPendingDatagrams())
{
QByteArray datagram;
datagram.resize(udpSocket->pendingDatagramSize());
udpSocket->readDatagram(datagram.data(),datagram.size());
QDataStream in(&datagram,QIODevice::ReadOnly);
int msgType;
in >> msgType;
QString usrName,ipAddr,msg;
//QTime DataTime=QTime::currentTime();
//QString time=DataTime.toString("yyyy-MM-dd hh:mm:ss");
QString time=QDateTime::currentDateTime().toString("yyyy-MM-dd hh:mm:ss");
switch(msgType)
{
case Msg:
in >> usrName >> ipAddr >> msg;
ui->messagebox->setTextColor(Qt::blue);
ui->messagebox->setCurrentFont(QFont("Times New Roman",12));
ui->messagebox->append("["+usrName+"]"+time);
ui->messagebox->append(msg);
break;
case UsrEnter:
in >> usrName >>ipAddr;
usrEnter(usrName,ipAddr);
break;
case UsrLeft:
in >> usrName;
usrLeft(usrName,time);
break;
case FileName: {break;}
case Refuse: {break;}
}
}
}
//用户进入
void Widget::usrEnter(QString usrname, QString ipaddr)
{
bool isEmpty=ui->usrTblWidget->findItems(usrname,Qt::MatchExactly).isEmpty();
if(isEmpty){
QTableWidgetItem *usr = new QTableWidgetItem(usrname);
QTableWidgetItem *ip = new QTableWidgetItem(ipaddr);
ui->usrTblWidget->insertRow(0);
ui->usrTblWidget->setItem(0,0,usr);
ui->usrTblWidget->setItem(0,1,ip);
ui->messagebox->setTextColor(Qt::gray);
ui->messagebox->setCurrentFont(QFont("Times New Roman",10));
ui->messagebox->append(usrname+"上线!\n");
ui->usrNumLbl->setText(tr("在线人数:%1").arg(ui->usrTblWidget->rowCount()));
sndMsg(UsrEnter); //使其他端点用户在列表中加入该用户
}
//else
// ui->usrNumLbl->setText(tr("没有人!"));
}
//用户离开
void Widget::usrLeft(QString usrname, QString time)
{
int rowNum = ui->usrTblWidget->findItems(usrname,Qt::MatchExactly).first()->row();
ui->usrTblWidget->removeRow(rowNum);
ui->messagebox->setTextColor(Qt::gray);
ui->messagebox->setCurrentFont(QFont("Times New Roman",10));
ui->messagebox->append(tr("%1于%2离开!").arg(usrname).arg(time));
ui->usrNumLbl->setText(tr("在线人数:%1").arg(ui->usrTblWidget->rowCount()));
}
QString Widget::getIP()
{
QList<QHostAddress>list = QNetworkInterface::allAddresses();
foreach (QHostAddress addr,list) {
if(addr.protocol()==QAbstractSocket::IPv4Protocol)
return addr.toString();
}
return 0;
}
QString Widget::getUsr()
{
return uName;
}
//获取文本框内容
QString Widget::getMsg()
{
QString msg=ui->send_message->toHtml();
ui->send_message->clear();
ui->send_message->setFocus();
//接收正常
//ui->messagebox->append(msg);
return msg;
}
//按发送键发送
void Widget::on_sendBtn_clicked()
{
sndMsg(Msg);
}
void Widget::on_exitBtn_clicked()
{
close();
}
//用户离开
void Widget::closeEvent(QCloseEvent *e)
{
sndMsg(UsrLeft); //发送广播
QWidget::closeEvent(e); //使其他端点在其用户列表中删除该用户
}