Course for This Assignment | EE308FZ |
---|
Team Name | Heha, two generals |
Team Member 1 | Chen Zhanming 832202117 |
Team Member 2 | Gao Liqin 832202126 |
Assignment Requirements | Extreme Programming |
Objectives of This Assignment | 1.Bookmark contacts 2.Add multiple contact methods 3.Import and Export |
目录
- 1.Introduction
- Project Background
- Project objectives
- Team work
- Team division of labor
- 2.Project address
- 3.Functional implementation ideas
- Addition, deletion, modification, and search of user information
- User Information Paging Query and Search
- Collection function
- Excel Import and Export Functionality
- Backend Database Interaction and Data Validation
- Error Handling and Response
- 4. GitHub submission log
- 5. Running video and function introduction
- 6. Team division of labor and contribution evaluation
- Division of labor among team members
- Contribution ratio evaluation
- 7. Difficulties encountered and solutions
- Data synchronization issues
- Data formatting issues during Excel import
- 8.PSP form
- Chen Zhanming's PSP form
- Gao Liqin's PSP form
- 9. Project Summary
- Project Background and Objectives
- Team division of labor and contribution
- Difficulties encountered and solutions
- Work Summary
1.Introduction
Project Background
In today's rapidly developing information age, contact management systems have become an indispensable part of daily work and life. With the diversification of communication methods and the increase in the number of contacts, how to effectively and conveniently manage contact information is an important issue. Traditional contact management methods often suffer from the following issues: inability to flexibly tag frequently used contacts, inability to provide multiple contact methods for a single contact, lack of import and export functionality, and low operational efficiency. Therefore, developing a contact management system that integrates multiple functions can not only improve efficiency, but also enhance the user experience.
Project objectives
The goal of this project is to design and implement a web-based contact management system with the following core functions:
- Contact bookmark function: Users can mark certain contacts as "important" or "frequently used" for easy access in the future.
- Support multiple contact methods: Each contact can be associated with multiple contact methods, such as phone numbers, emails, social media accounts, etc., to ensure the comprehensiveness of information.
- Import and Export Function: Implement the import and export of contact information, allowing users to easily import data into the system or export system data as Excel files for backup or migration.
- Web deployment (optional): Deploy the project to a cloud server (such as Huawei Cloud, Alibaba Cloud, etc.) so that the system can be accessed and used online anytime, anywhere.
Through these features, this system can not only help users efficiently manage contacts, but also provide convenient cross platform data migration and backup functions.
Team work
This project was jointly completed by two members. Despite the small size of the team, we successfully achieved all the goals of the project through close cooperation and reasonable division of labor. Each member was fully involved in the design, coding, testing, and deployment of the project. During the team collaboration, we fully utilized GitHub for version management and ensured collaborative development of the code.
Through clear division of labor and efficient communication, we completed all tasks of the project within the specified time. During this process, we solved multiple technical challenges and ensured the smooth completion of the project through mutual support.
Team division of labor
Due to the small number of team members, we adopted a highly collaborative approach in terms of division of labor. Each member participated in the development and implementation of multiple modules of the project, ensuring the smooth progress of the project. Specifically, Chen Zhanming was responsible for the design and implementation of bookmark functionality, as well as participating in web deployment work; Gao Liqin was mainly responsible for the implementation of import and export functions, and assisted in the design of the front-end page.
2.Project address
- GitHub repository address:
3.Functional implementation ideas
This project has implemented a user information management system, which mainly includes the following functional modules: adding, deleting, modifying, and querying user information, bookmarking functions, and importing and exporting Excel files. For the convenience of front-end operations, all these functions are provided through RESTful APIs, and the front-end interacts with the back-end through Vue.js and Element UI. The following are detailed implementation ideas for each functional module:
The management of user information is a fundamental function of the system, including adding users, updating user information, deleting users, and querying user information.
- New user: The front-end submits user information through a form, and the back-end receives the request and saves the user information to the database. The specific operation is handled by the add() method annotated with @ PostMapping, using userService. save (user) to save the data to the database.
- Update user information: Use the @ PutMapping annotation's update() method to process update requests. The front-end will submit the modified user data, and the back-end will query the database based on the user ID and update the corresponding fields. This operation is similar to the add operation, using userService. save (user) to perform the update.
- Delete user: The deletion operation is implemented through the delete() method of @ DeleteMapping ("/{id}") annotation. After receiving the user ID, call userService. del (id) to delete the records in the database. After the deletion operation is successful, return a message saying 'deletion successful'.
- Query user information: Using the findById() method annotated with @ GetMapping ("/{id}"), after receiving the user ID, query the database and return the user's detailed information. If the user does not exist, an error message will be returned.
In order to avoid loading a large amount of data at once, the system supports pagination to query user information and fuzzy search by username.
- Paging query: The findPage() method takes page numbers and page sizes as parameters, and uses PageRequest and Sort to create paging requests. Use the userDao. findNameLike() method to query the database and return paginated data.
- Fuzzy search: The front-end provides the function of searching by username. Users can enter their username in the search box, and the back-end performs fuzzy queries based on their username. Through the @ GetMapping ("/page") interface, the frontend passes the name parameter for querying. UserDao. findNameLike() uses the like statement in SQL to match usernames.
Collection function
The bookmarking function allows users to mark certain contacts as "bookmarked" for quick access. The system supports user bookmarking and unsubscribing operations.
- Favorite User: The front-end triggers the 'toggleCollect (user)' method by clicking the 'Favorite' button, which updates the user's collection status. The backend saves the updated user information through 'userService. save (updatedUser)'. The user's collection status is represented by the field 'collect', with a value of 'yes' indicating collected and a value of' no 'indicating not collected.
- View Favorite Users: When the user clicks the "Favorite User List" button, the front-end requests the data of the bookmarked user from the back-end through the
loadCollect()
method. The backend uses the userService. findByCollect()
method to query the collected users in the database based on the collect
field being 'yes', and returns them in pagination.
Excel Import and Export Functionality
In order to facilitate users to manage contact information in bulk, the system provides Excel import and export functions.
- Export User Information: The front-end exports the current user information as an Excel file by clicking the "Export Excel" button. The front-end uses the 'xlsx' library to convert user data into Excel format. The exported data includes information such as username, age, gender, email, phone number, and collection status. The backend did not process the export, only passed the existing paginated data to the frontend, which saved the data as an Excel file using 'XLSX. riteFile()'.
- Import User Information: After the user uploads an Excel file, the front-end will use the 'xlsx' library to parse the file content, convert the data to JSON format, and then send it to the back-end through the 'importData()' method. The backend processes the imported data using the
userService. importUser()
method to determine if the same user already exists in the data (by checking the email field). If the user already exists, update their information; If the user is a new user, insert it into the database. - Import Data Merge: When importing data, the backend checks whether each piece of data already exists (determined by email) and updates the existing data to avoid duplicate data insertion.
Backend Database Interaction and Data Validation
The backend uses Spring Data JPA to implement database interaction, mainly involving the following parts:
- User data saving and deletion: Use the 'save()' and 'deleteById()' methods provided by JpaRepository to perform data addition and deletion operations` UserService. save (user) will save user data to the database, while userService. del (id) will delete user records based on the user ID.
- Paging Query and Sorting: When querying user information, the
findPage()
and findByCollect()
methods implement paging and sorting functions through PageRequest
and ` Sort `` FindPage() supports fuzzy queries by username, while findByCollect() queries the collected users based on the 'collect' field. - Batch Import: The
importUser()
method receives a list of users and imports data into the database one by one. The method internally checks whether the email already exists through 'userDao. findByEmail()', updates the existing record if it exists, and inserts a new record if it does not exist.
Error Handling and Response
Each API interface has undergone error handling and response encapsulation:
- Successful Response: Use the
Result. success()
method to return a response indicating the success of the operation, including a success message. - Error Response: For user not found or other errors, use 'Result.Error()' to return the error code and message, ensuring that the frontend receives detailed error information.
4. GitHub submission log
- Screenshot of submission times:

- GaoLiqin:5 times
- ChenZhanming:4 times
5. Running video and function introduction
Running video:
Collect
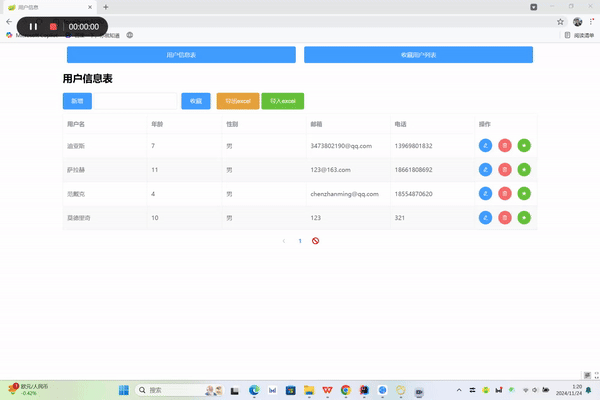
Export Excel
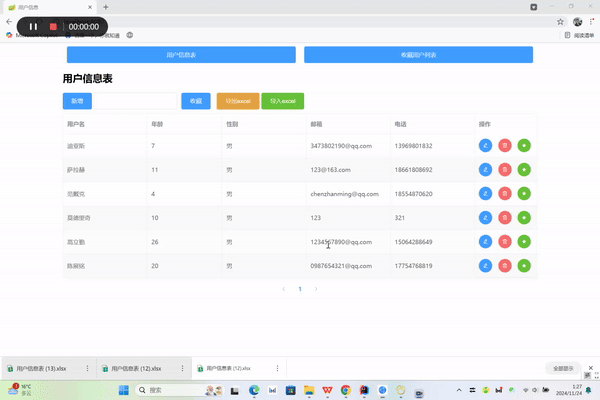
Insert Excel
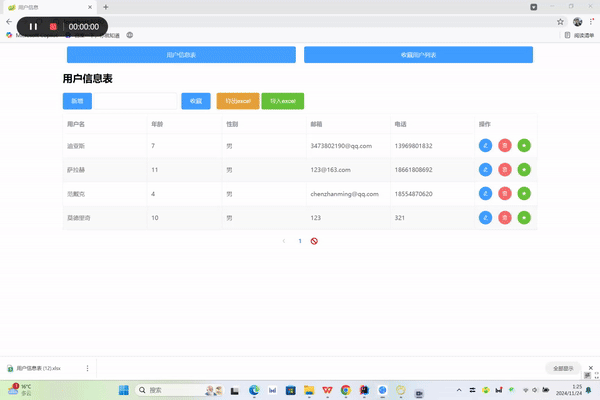
Function Introduction:
- There are two buttons at the top of the page: User Information Table and Favorite User List. Click the User Information Table to view the users in the address book, and click the Favorite User button to view the favorite user information. Click the Add button to add a contact, and you can add two contact methods: email and phone. After adding, the user information will appear in the User Information Table. Click the star button on the right side of the user information to favorite the contact, and the favorite contact information will be stored in the Favorite User List. Click the Export Excel button to export the information in the User Information Table to Excel, and click the Import Excel button to choose to import Excel into the address book. The user information will appear in the User Information Table
6. Team division of labor and contribution evaluation
In this project, team members worked closely together to complete the design, development, and implementation of the project. Due to the small number of team members, each member actively participated in the implementation of multiple functional modules and system testing. The following is the specific division of labor within the team and the evaluation of each member's contribution.
Division of labor among team members
- Chen Zhanming:
- Responsible for functionality: Responsible for implementing user information display and management, as well as bookmarking functions.
- Specific work:
- Designed and implemented an el table for displaying user information, and successfully integrated pagination and search functions, enabling users to conveniently browse, filter, and manage user data.
- Responsible for implementing the bookmarking function, users can bookmark or unlink users by clicking the button. After each operation, the system will update the user's "Favorites" field based on their favorite status.
- Implemented the display of the list of users who have been bookmarked, by calling the backend interface from the front-end to display the list of users who have been bookmarked.
- Gao Liqin:
- Responsible for functionality: Responsible for implementing Excel import and export functions, and supporting batch import of user data.
- Specific work:
- The Excel export function has been completed, allowing users to export their current user data as an Excel file. The XLSX library has been used to process the data export, ensuring the correct format of the data.
- Implemented Excel import function, allowing users to upload Excel files and import user data in batches into the system. Use FileReader and XLSX libraries to parse Excel files and submit the data to the backend for processing.
- Assisted in the design of pagination and search functions, ensuring efficient loading of user data and supporting fuzzy queries by name.
Contribution ratio evaluation
- Chen Zhanming's Contribution: Mainly responsible for the implementation of user information display and bookmarking functions, ensuring that the system can display user information and provide bookmarking operations. The front-end functions responsible by member A are crucial for user experience, especially the display and dynamic updates of user favorites lists.
- Contribution of Gao Liqin: Mainly responsible for the implementation of Excel import and export functions. Through this feature, users can easily import and export data in batches, greatly improving the efficiency of data management. In addition, member B also assisted in completing pagination and search functions, improving the flexibility and availability of the system.
Due to the important role played by each member in the project and the active collaboration between both parties, the final implementation of the project was successfully completed. Therefore, the contribution ratio of the team is evaluated as 50%: 50%.
7. Difficulties encountered and solutions
During the development process of this project, although the team consisted of only two members, we still encountered some technical issues and challenges. The following are the main difficulties we encountered and their solutions.
Data synchronization issues
- Problem description: In the data exchange between the front-end and back-end, we have found that sometimes the results of data updates are not immediately reflected in the front-end interface. For example, after updating user information, the front-end table still displays old data, which prevents users from seeing the latest modifications.
- Solution: To address this issue, we ensure that the interface is called again to refresh the table data after each data update (such as adding, modifying, or deleting users). After each operation, the 'loadTable()' method is triggered to retrieve the latest data from the backend and update the data in the frontend table through 'this. page. content'. This ensures that the frontend always displays the latest data in the database.
$.ajax({
url: '/user',
type: 'post',
contentType: 'application/json',
data: JSON.stringify(this.form)
}).then(() => {
this.dialogVisible = false;
this.loadTable(1);
});
- Problem description: When implementing the Excel file import function, we encountered issues with processing data in different formats. Especially when the field names in the Excel files uploaded by users are inconsistent with the backend database fields, the data cannot be mapped correctly during import, and some fields are not stored correctly.
- Solution: To solve the field mapping problem, we have performed field mapping on Excel data in the front-end. After reading Excel files through the xlsx library, use custom mapping rules to convert field names (such as "username") in Excel to the field names required by the backend database (such as "name"). Then transfer the converted data to the backend for storage.
const mappedData = jsonData.map(item => ({
name: item["用户名"],
age: item["年龄"],
sex: item["性别"],
email: item["邮箱"],
phone: item["电话"],
collect: item["收藏"]
}));
Through this field mapping, we ensure the correctness of data when importing from Excel, while avoiding issues of data loss and formatting errors.
Stage | Task Description | Time Spent |
---|
Plan | Determine the implementation scheme for user information display and collection functions, plan front-end design and data interaction | 2 hours |
Design | Design a user information display table (el table), establish the interaction logic between the bookmark button and the bookmark status | 3 hours |
Encoding | Implement user information display table, pagination and search functions, interaction of favorites button, and front-end and back-end interface integration | 8 hours |
Test | Test the interaction effect between the user information table and the bookmarking function to ensure that the data can be displayed and updated correctly | 3 hours |
Debugging and Optimization | Fix display issues, optimize the refresh of favorites function, and ensure data synchronization | 2 hours |
Documentation and Summary | Write partial functional descriptions, update GitHub project documentation, participate in project summary and reflection | 2 hours |
Total | | 20 hours |
Stage | Task Description | Time Spent |
---|
Plan | Participate in determining the implementation plan for Excel import and export functions, and plan the interaction between the front-end and back-end | 2 hours |
Design | Design the UI for Excel import and export functions, determine the data format and backend interaction plan | 3 hours |
Encoding | Implement Excel export function, use xlsx library to process file data; Implement Excel import function, parse files and map fields | 9 hours |
Test the import and export function of Excel to ensure that the data format is correct and that the data can be imported and displayed correctly | 3 hours | |
Debugging and Optimization | Fix data mapping issues during import process, optimize file upload and data display | 2 hours |
Document and Summary | Write Excel import and export function documents, participate in project summary and reflection | 2 hours |
Total | | 21 hours |
Summary:
- Chen Zhanming was mainly responsible for the front-end development, interaction design, and front-end and back-end integration of user information display and collection functions, which took a total of 20 hours.
- Gao Liqin was responsible for the implementation of Excel import and export functions, involving front-end UI design, back-end interface implementation, data processing, and testing, which took a total of 21 hours.
Total working hours: 41 hours, with A and B contributing equally, distributed at a ratio of 50%: 50%.
9. Project Summary
Project Background and Objectives
This project aims to implement a contact management system, which includes multiple core functions:
- User Information Display and Management: Display basic user information and support pagination and search functions.
- Favorite function: Allow users to tag and bookmark important contacts for easy access in the future.
- Excel import and export: Implement batch import and export of user information to improve the efficiency of data management.
The implementation of the project relies on close collaboration between the front-end and back-end, with the front-end responsible for user interface and interaction, and the back-end responsible for data storage and processing.
Team division of labor and contribution
This project is jointly completed by two members, with reasonable task allocation to ensure that each member can contribute in their respective fields.
- Chen Zhanming is responsible for the implementation of user information display and collection functions, mainly focusing on the front-end table display, pagination, search functions, and the switching of collection status. It took 20 hours.
- Gao Liqin was responsible for implementing the import and export functions of Excel, handling tasks such as file format parsing, data mapping, file uploading, and backend interface integration. It took 21 hours.
Two members each took on important responsibilities in the project, with a contribution ratio of 50%: 50%, and the workload was basically balanced.
Difficulties encountered and solutions
During the project development process, the team encountered some technical challenges, mainly including the following aspects:
- Data synchronization issue: After updating user data, the front-end interface did not refresh and display the latest data in a timely manner. To address this issue, we ensure that the refresh interface is called after each data operation to ensure that the data displayed by the frontend is always consistent with the backend.
- Data consistency issue with the bookmarking function: After the bookmarking status is updated, the front-end interface fails to reflect the latest status in the database in a timely manner. We successfully resolved this issue by reloading the table data and refreshing the favorites list after the bookmarking operation.
- Data format issue during Excel import: Due to the inconsistency between field names in Excel and backend fields, data mapping problems occur during import. By mapping fields in the front-end, ensure that data can be stored correctly in the database.
- Data merging issue during Excel import: To avoid duplicate data during the import process, we checked and updated the imported Excel data to ensure that updates were only performed when necessary, avoiding unnecessary database operations.
These difficulties were successfully overcome through close collaboration among team members and flexible solutions, ensuring the smooth progress of the project.
Work Summary
Through this project development, the team not only successfully implemented various functions of the system, but also improved their technical and teamwork abilities by solving practical problems. Within a limited time, team members completed all functional development tasks through reasonable division of labor and efficient collaboration. Especially in the implementation of Excel import and export functions and collection functions, we have accumulated valuable experience and learned how to handle complex data interaction and file processing tasks.
The development of this project not only improved our technical level, but also deepened our understanding of team collaboration and project management, especially how to effectively allocate tasks, solve problems, and ensure project completion on time in a small team environment.