以下是生成公钥和私钥的代码,不知为何,生成的公钥和私钥与标准的位数相差甚远,但是参数设定与标准设定相同,请大佬赐教,有急用,谢谢啦

#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <time.h>
#include <openssl/bn.h>
#include <openssl/ec.h>
#include <openssl/bio.h>
#include <openssl/x509.h>
#include <openssl/rand.h>
#include <openssl/evp.h>
#include <openssl/crypto.h>
#include <openssl/err.h>
#include <openssl/ecdsa.h>
#include <openssl/ecdh.h>
#include "sm3.h"
#include "sm2.h"
#define PRIVKEY "static unsigned char privkey[%d] = {"
#define PUBKEY "static const unsigned char pubkey[%d] = {"
#define ENDKEY "\n};\n"
#pragma comment(lib,"libeay32.lib")
#define ABORT do { \
fflush(stdout); \
fprintf(stderr, "%s:%d: ABORT\n", __FILE__, __LINE__); \
ERR_print_errors_fp(stderr); \
exit(1); \
} while (0)
static const char rnd_seed[] = "string to make the random number generator think it has entropy";
void BNPrintf(BIGNUM* bn)
{
char *p=NULL;
p=BN_bn2hex(bn);
printf("%s",p);
OPENSSL_free(p);
}
int main()
{
//生成摘要
int ilen = 3;
unsigned char output[32];
unsigned char digest[1388][32];
sm3_context ctx3;
BN_CTX *ctx = NULL;
BIGNUM *p, *a, *b;
EC_GROUP *group;
EC_POINT *P, *Q, *R;
BIGNUM *x, *y, *z;
EC_KEY *eckey = NULL;
unsigned char *signature = NULL;
int sig_len;
//FILE *fp;
char pubkey[341][5]={0};
char prikey[65][5]={0};
int i=0,cs=0,er=0;
int aa=0,bb=0,cc=0;
char sta[16][5]={"0000","0001","0010","0011","0100","0101","0110","0111","1000","1001","1010","1011","1100","1101","1110","1111"};
char all2sign[1388][72][2][5];/*72是由签名长度决定*/
int sta10[16]={0,1,2,3,4,5,6,7,8,9,10,11,12,13,14,15};
int all10sign[1388][72];
unsigned char qq[1000];
int longsig[1387];
//int ilen = 3;
//unsigned char output[32];
//sm3_context ctx;
FILE*tp; FILE*mp; FILE*np;
FILE *file;
char buf[1200];
int len2=0,j=0,k=0;
unsigned char array [1389][600];
unsigned char bufpri[341], bufpub[65];
unsigned char *pp;
int len;
int sl;
for(cs=0; cs < 1; cs++)
{CRYPTO_set_mem_debug_functions(0, 0, 0, 0, 0);
CRYPTO_mem_ctrl(CRYPTO_MEM_CHECK_ON);
ERR_load_crypto_strings();
RAND_seed(rnd_seed, sizeof rnd_seed);
/*SM2_Test_Vecotor();*/
//int len2=0,i=0,j=0,k=0;
//unsigned char array [1389][500];
CRYPTO_set_mem_debug_functions(0, 0, 0, 0, 0);
CRYPTO_mem_ctrl(CRYPTO_MEM_CHECK_ON);
ERR_load_crypto_strings();
RAND_seed(rnd_seed, sizeof rnd_seed); /* or BN_generate_prime may fail */
ctx = BN_CTX_new();
if (!ctx) ABORT;
/* Curve SM2 (Chinese National Algorithm) */
//http://www.oscca.gov.cn/News/201012/News_1197.htm
p = BN_new();
a = BN_new();
b = BN_new();
if (!p || !a || !b) ABORT;
group = EC_GROUP_new(EC_GFp_mont_method()); /* applications should use EC_GROUP_new_curve_GFp
* so that the library gets to choose the EC_METHOD */
if (!group) ABORT;
if (!BN_hex2bn(&p, "8542D69E4C044F18E8B92435BF6FF7DE457283915C45517D722EDB8B08F1DFC3")) ABORT;
if (1 != BN_is_prime_ex(p, BN_prime_checks, ctx, NULL)) ABORT;
if (!BN_hex2bn(&a, "787968B4FA32C3FD2417842E73BBFEFF2F3C848B6831D7E0EC65228B3937E498")) ABORT;
if (!BN_hex2bn(&b, "63E4C6D3B23B0C849CF84241484BFE48F61D59A5B16BA06E6E12D1DA27C5249A")) ABORT;
if (!EC_GROUP_set_curve_GFp(group, p, a, b, ctx)) ABORT;
P = EC_POINT_new(group);
Q = EC_POINT_new(group);
R = EC_POINT_new(group);
if (!P || !Q || !R) ABORT;
x = BN_new();
y = BN_new();
z = BN_new();
if (!x || !y || !z) ABORT;
// sm2 testing P256 Vetor
// p:8542D69E4C044F18E8B92435BF6FF7DE457283915C45517D722EDB8B08F1DFC3
// a:787968B4FA32C3FD2417842E73BBFEFF2F3C848B6831D7E0EC65228B3937E498
// b:63E4C6D3B23B0C849CF84241484BFE48F61D59A5B16BA06E6E12D1DA27C5249A
// xG 421DEBD61B62EAB6746434EBC3CC315E32220B3BADD50BDC4C4E6C147FEDD43D
// yG 0680512BCBB42C07D47349D2153B70C4E5D7FDFCBFA36EA1A85841B9E46E09A2
// n: 8542D69E4C044F18E8B92435BF6FF7DD297720630485628D5AE74EE7C32E79B7
if (!BN_hex2bn(&x, "421DEBD61B62EAB6746434EBC3CC315E32220B3BADD50BDC4C4E6C147FEDD43D")) ABORT;
if (!EC_POINT_set_compressed_coordinates_GFp(group, P, x, 0, ctx)) ABORT;
if (!EC_POINT_is_on_curve(group, P, ctx)) ABORT;
if (!BN_hex2bn(&z, "8542D69E4C044F18E8B92435BF6FF7DD297720630485628D5AE74EE7C32E79B7")) ABORT;
if (!EC_GROUP_set_generator(group, P, z, BN_value_one())) ABORT;
if (!EC_POINT_get_affine_coordinates_GFp(group, P, x, y, ctx)) ABORT;
fprintf(stdout, "\nChinese sm2 algorithm test -- Generator:\n x = 0x");
BNPrintf(x);
fprintf(stdout, "\n y = 0x");
BNPrintf( y);
fprintf(stdout, "\n");
/* G_y value taken from the standard: */
if (!BN_hex2bn(&z, "0680512BCBB42C07D47349D2153B70C4E5D7FDFCBFA36EA1A85841B9E46E09A2")) ABORT;
if (0 != BN_cmp(y, z)) ABORT;
fprintf(stdout, "verify degree ...");
if (EC_GROUP_get_degree(group) != 256) ABORT;
fprintf(stdout, " ok\n");
fprintf(stdout, "verify group order ...");
fflush(stdout);
if (!EC_GROUP_get_order(group, z, ctx)) ABORT;
if (!EC_GROUP_precompute_mult(group, ctx)) ABORT;
if (!EC_POINT_mul(group, Q, z, NULL, NULL, ctx)) ABORT;
if (!EC_POINT_is_at_infinity(group, Q)) ABORT;
fflush(stdout);
fprintf(stdout, " ok\n");
//testing ECDSA for SM2
/* create new ecdsa key */
if ((eckey = EC_KEY_new()) == NULL)
goto builtin_err;
if (EC_KEY_set_group(eckey, group) == 0)
{
fprintf(stdout," failed\n");
goto builtin_err;
}
/* create key */
if (!EC_KEY_generate_key(eckey))
{
fprintf(stdout," failed\n");
goto builtin_err;
}
pp = bufpri;
len =i2d_ECPrivateKey(eckey,&pp);
if (!len)
{
printf("Error:i2d_SM2PrivateKey()\n");
EC_KEY_free(eckey);
return -1;
}
printf(PRIVKEY,len);
for (i=0; i<len; i++)
{
if ( !(i % 10) )
printf("\n");
if(i==len-1)
printf("0x%02X ",bufpri[i]);
else
printf("0x%02X , ",bufpri[i]);
}
printf(ENDKEY);
/* check key */
if (!EC_KEY_check_key(eckey))
{
fprintf(stdout," failed\n");
goto builtin_err;
}
pp = bufpub;
len = i2o_ECPublicKey(eckey,&pp);
if (!len)
{
printf("Error:i2o_SM2PublicKey()\n");
EC_KEY_free(eckey);
return -1;
}
printf(PUBKEY,len);
for (i=0; i<len; i++)
{
if ( !(i % 10) )
printf("\n");
if(i==len-1)
printf("0x%02X ",bufpub[i]);
else
printf("0x%02X , ",bufpub[i]);
}
printf(ENDKEY);
//EC_KEY_free(eckey);
EC_KEY_free(eckey);
eckey = NULL;
builtin_err:
EC_POINT_free(P);
EC_POINT_free(Q);
EC_POINT_free(R);
EC_GROUP_free(group);
BN_CTX_free(ctx);
CRYPTO_cleanup_all_ex_data();
ERR_free_strings();
ERR_remove_state(0);
CRYPTO_mem_leaks_fp(stderr);
};
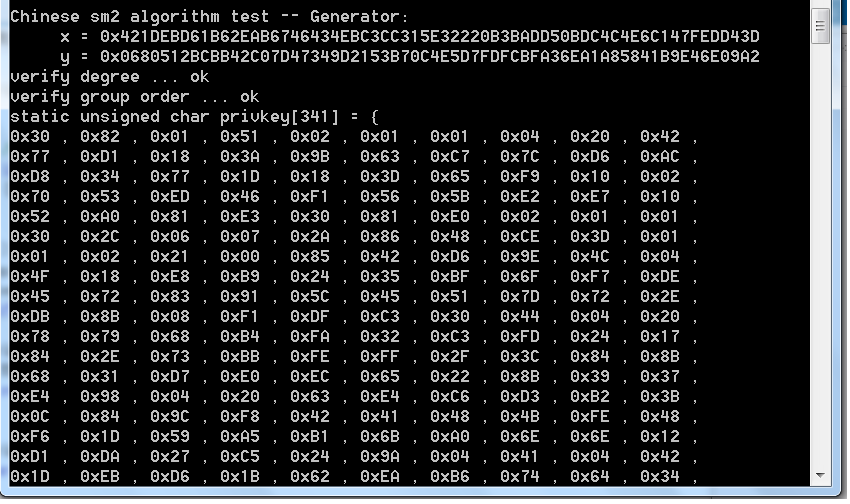
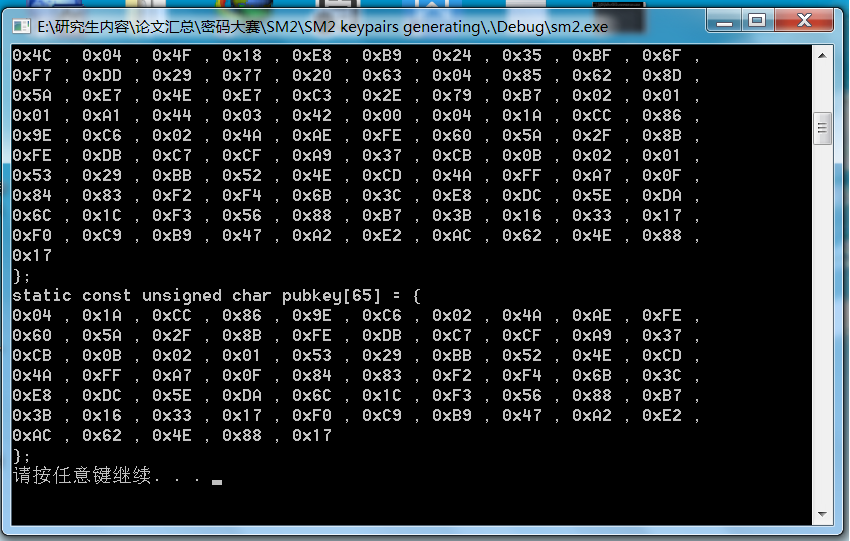